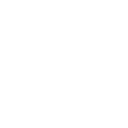
0
XTRF Groovy Macro - How to compare numerical values with non-numeric value 'Hyphens'
How to check in XTRF Macros when value is a whole number or with decimal places and/or non-numeric values exists such as hyphens.
Example 1: Quote Value can be 0
Example 2: Quote Value can be 0.00
Example 3: Quote Value can be -
Customer support service by UserEcho
Dear Burg,
This is a plain java question that you have. I think the best thing you could do is to look into Google and get some examples there such as these https://www.baeldung.com/java-check-string-number
As example for your specific problem, this will give you a push in the right direction:
The important part is that the "-" is null in most places. It is just a visual placeholder for the front end to mark that there's "no value" in a given field. I'm not sure which field exactly you are talking about, but keep your eyes peeled :)
That being said, here's the answer to your question:
Checking if the given value is a specific type
That's very simple; you just need to use the instanceof operator.
Comparing two numbers
The way to go is to use type casting as-is and handle the potential exception:
Another way is to use the built-in Number class and/or the `instanceof` operator and type casting if you don't want to make the comparison if the type doesn't match: